๐ก About Command Pattern
์ปค๋งจ๋ ํจํด์ ์ฃผ์ด์ง ๊ธฐ๋ฅ๋ค์ ์บก์ํํจ์ผ๋ก์จ ์๋ก ๋ค๋ฅธ ์์ฒญ๋ค์ ์คํํ ์ ์๋๋ก ํ๋ ํจํด์ด๋ค.
๊ฐ๋จํ ๋งํ์๋ฉด ๊ฐ์ฒด์ ํ์(๋ฉ์๋)๋ฅผ ํด๋์ค๋ก ๋ง๋ค์ด ์บก์ํํ๋ ํจํด์ธ๋ฐ, A ๊ฐ์ฒด์์ B ๊ฐ์ฒด์ ๋ฉ์๋๋ฅผ ์คํํ๋ ค๋ฉด B ๊ฐ์ฒด๋ฅผ ์ฐธ์กฐํ๊ณ ์์ด์ผํ๋ ์์กด์ฑ์ด ๋ฐ์ํ๋ค.
→ ์ปค๋งจ๋ ํจํด์ ์ ์ฉํ๋ฉด ์ด๋ฐ ์์กด์ฑ์ ์ ๊ฑฐํ๊ณ , ๊ธฐ๋ฅ๋ค์ ์์ , ์ถ๊ฐํ ๋ ํ์ฅ์ฑ๊ณผ ์ ์ฐ์ฑ์ด ์ข์์ง๋ค.
๐ก Command Pattern Structure
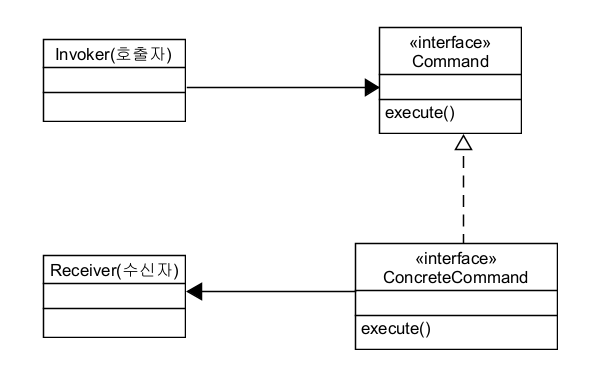
Invoker
→ ๊ธฐ๋ฅ์ ์คํ์ ์์ฒญํ๋ ํธ์ถ์ ํด๋์ค
Command
→ ์คํ๋ ๊ธฐ๋ฅ์ ๋ํ ์ธํฐํ์ด์ค
- ์คํ๋ ๊ธฐ๋ฅ์ execute ๋ฉ์๋๋ก ๊ตฌํ
ConcreteCommand
→ Command ์ธํฐํ์ด์ค ๊ตฌํ
Receiver
→ ConcreteCommand์์ execute ๋ฉ์๋๋ฅผ ๊ตฌํํ ๋ ํ์ํ ํด๋์ค
๐ก Practice Command Pattern
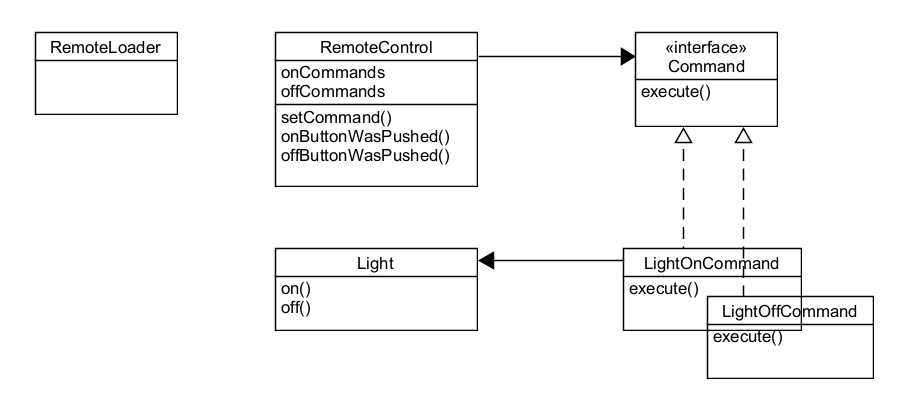
์ฃผ์ด์ง ๋ค์ด์ด๊ทธ๋จ๊ณผ ๊ฐ์ด RemoteControl์์ Light๋ฅผ on/offํ๋ ๋ฑ์ ์คํ์ ์์ฒญํ๊ณ , ๊ทธ ๊ณผ์ ์ด Command๋ฅผ ์์๋ฐ์ LightOnCommand์์ ๊ตฌํ๋ ํ๋ก๊ทธ๋จ์ ๋ง๋ค์ด๋ณด์.
- Command
package Command;
public interface Command {
public void execute();
public void undo();
}
- Light
public class Light {
String location = "";
public Light(String location) {
this.location = location;
}
public void on() {
System.out.println(location + " light is on");
}
public void off() {
System.out.println(location + " light is off");
}
}
- LightOnCommand
Light๊ฐ์ฒด๋ฅผ ๊ฐ์ ธ์์ Light์ ๋ฉ์๋๋ฅผ ์ฌ์ฉํจ์ผ๋ก์จ LightOnCommand์์๋ execute() ๋ฉ์๋๋ง์ ์ฌ์ฉํ ์ ์๋ค. ↔ LightOnCommand์์ Light์ ๋ฉ์๋๋ฅผ ์คํํ๋๋ฐ ์์กด์ฑ์ ์ ๊ฑฐํ๋ค.
package Command;
public class LightOnCommand implements Command{
Light light;
public LightOnCommand(Light light){
this.light = light;
}
public void execute(){
light.on();
}
@Override
public void undo() {
}
}
- LightOffCommand
package Command;
public class LightOffCommand implements Command{
Light light;
public LightOffCommand(Light light){
this.light = light;
}
public void execute(){
light.on();
}
}
- RemoteControl โญ
Command๊ฐ์ฒด ๋ฐฐ์ด์ ์ ์ธํ์ฌ main์์ ์ธ๋ฑ์ค๋ฅผ ์ ๋ ฅํ๋ฉด ๊ฐ์ฒด๋ฅผ ์์ฑํ๊ณ , ์ํํ ๊ฐ์ฒด๋ณ๋ก setCommand๊น์ง ํ ์ ์๋๋ก ํ๋ค.
์๋ฅผ๋ค๋ฉด,RemoteControl ๊ฐ์ฒด๋ฅผ ์์ฑํ ํ, lightCommand์ on์ executeํ๋ค
package Command;
public class RemoteControl {
// on/off ๋ฉ์๋๊ฐ ๊ตฌํ๋ 7๊ฐ์ ๊ฐ์ฒด๊ฐ ์๋ ์ํฉ ex) Light, TV
Command[] onCommand = new Command[7];
Command[] offCommand = new Command[7];
Command undoCommand = new NullCommand();
// NullCommand -> Null๋ก setting
RemoteControl(){
for(int i = 0; i < 7; i++){
onCommand[i] = new NullCommand();
offCommand[i] = new NullCommand();
}
}
// ๊ตฌํ๋ ๋ฉ์๋๊ฐ ๋ชจ๋ on/off๋ก ๊ฐ์ -> command array๋ก ๊ตฌํ ๊ฐ๋ฅ
public void setOnCommand(int num, Command myCommand){
onCommand[num] = myCommand;
}
public void setOffCommand(int num, Command myCommand){
offCommand[num] = myCommand;
}
public void onButtonWasPushed(int num) {
// button1 ๋๋ ธ์ ๋ ์ฝ๋
// Light myLight = new Light("Living Room");
// myLight.on();
// command1on.execute();
onCommand[num].execute();
}
public void offButtonWasPushed(int num) {
// myLight๋ฅผ ์์์ผ ํค๊ณ ๋ ์ ์๋ ์ํฉ
// <-> ๋ฆฌ๋ชจ์ปจ์ ์๋ํ ๋ ์ด๋ค ๊ฐ์ฒด๋ฅผ ๋ง๋ค์ด์ผํ ์ง ๋ค ์์์ผํ๋ค
// myLight.off();
offCommand[num].execute();
}
public String toString() {
StringBuffer stringBuff = new StringBuffer();
stringBuff.append("\n------ Remote Control -------\n");
return stringBuff.toString();
}
}
- Main
package Command;
public class Main {
// RemoteController์ ๊ธฐ๋ฅ๋ค์ ๋ชจ๋ ๋ฐ์ผ๋ก ๋นผ๋ฒ๋ฆฐ๊ฒ!
public static void main(String[] args) {
Light livingRoomLight = new Light("Living Room");
Light bedRoomLight = new Light("Bed Room");
TV myTV = new TV("Living Room");
RemoteControl myRemote = new RemoteControl();
// Setting ํ๊ณ
myRemote.setOnCommand(0, new LightOnCommand(livingRoomLight));
myRemote.setOnCommand(1, new TVOnCommand(myTV));
myRemote.setOffCommand(1, new TVOffCommand(myTV));
myRemote.setOnCommand(5, new enterCommand(livingRoomLight, myTV));
// ๋ฒํผ์ ๋๋ฅด๋๊ฒ
myRemote.onButtonWasPushed(0);
myRemote.onButtonWasPushed(1);
myRemote.offButtonWasPushed(0);
myRemote.onButtonWasPushed(5);
}
}
- LivingRoom์ ๋จํ๋ฅผ ์ผ๊ณ ์ถ๋ค๋ฉด
- remoteControl ๊ฐ์ฒด ์์ฑ
- Light ๊ฐ์ฒด ์์ฑ
- remoteControl์ setOnCommand ๋ฉ์๋๋ฅผ ํตํด Light์ ํด๋นํ๋ ๊ฐ์ฒด๋ก setting
- remoteControl์ onButtonPushed ๋ฉ์๋ ํธ์ถ
- TV ์ ์์ ๋๊ณ ์ถ๋ค๋ฉด
- ์ด๋ฏธ ์์ฑ๋ remoteControl๊ฐ์ฒด ์ฌ์ฉ ๊ฐ๋ฅ
- TV ๊ฐ์ฒด ์์ฑ
- remoteControl์ setOffCommand ๋ฉ์๋๋ฅผ ํตํด TV์ ํด๋นํ๋ ๊ฐ์ฒด๋ก setting
- remoteControl์ offButtonPushed ๋ฉ์๋ ํธ์ถ
์ด๋ฐ์์ผ๋ก ์ปค๋งจ๋ ํจํด์ ์ด์ฉํ๋ฉด on/off button๊ณผ ๊ฐ์ด ๊ฒน์น๋ ๋์๋ค์ ์ฝ๋๋ฅผ ๋ณ๊ฒฝํ์ง ์์ผ๋ฉด์ ๋ค์ํ ๊ฐ์ฒด์ ๋์์ ๊ตฌํํ ์ ์๊ฒ ๋๋ค๐ค
์ฐธ๊ณ
[Design Pattern] ์ปค๋งจ๋ ํจํด์ด๋ - Heee's Development Blog (gmlwjd9405.github.io)
'Software > Design Pattern' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[Design Pattern] Adapter Pattern (0) | 2021.11.23 |
---|---|
[Design Pattern] Observer Pattern (2) | 2021.10.08 |