๐ก About Adapter Pattern
Adapter Pattern์ ์ธํฐํ์ด์ค์ ์ธํฐํ์ด์ค๊ฐ์ ํธํ์ฑ ๋ฌธ์ ๋ฅผ ํด๊ฒฐํด์ฃผ๋ ํจํด์ด๋ค. ์ฝ์ผํธ์ ์ฌ์ฉํ๋ ๊ทธ ์ด๋ํฐ์ ์ญํ ๊ณผ ๋์ผ!
ํธํ๋์ง ์๋ ์ธํฐํ์ด์ค๋ฅผ ์ฌ์ฉํ๋ ์ฝ๋๋ค์ Adapter Class๋ฅผ ๋ง๋ค์ด ํ์ฉํ ์ ์๋ค.
- My existing system → Adapter → Vendor class
- Object Adapter์ Class Adapter๋ก ๊ตฌํ๋ฐฉ๋ฒ์ด ๋๋์ด์ง๋ค.
- ์ธํฐํ์ด์ค๊ฐ ํธํ๋์ง ์๋ ๋ฌธ์ ๊ฐ ๋ฐ์ํด๋ Adapter Pattern์ ํตํด ๊ธฐ์กด์ ์ฝ๋๋ฅผ ์์ ํ์ง ์๊ณ ๋ ๋ฌธ์ ๋ฅผ ํด๊ฒฐํ ์ ์๋ค.
๐ก Adapter Pattern Structure
Object Adapter Pattern
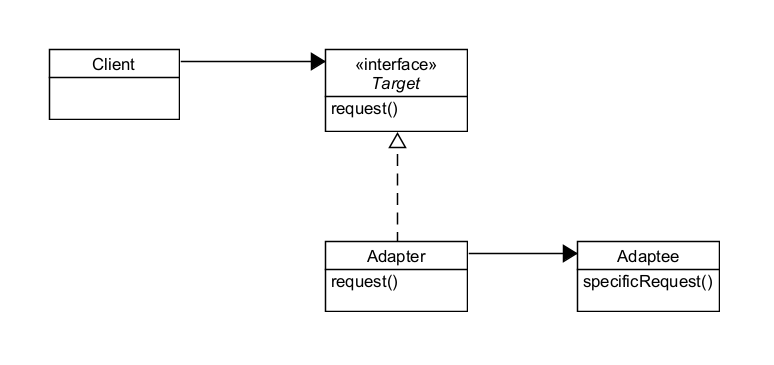
Client
→ Target Interface๋ฅผ ์๊ตฌํ๋ ํด๋์ค
Adaptee
→ ์ ์ฉํ ์ธ๋ถ์์คํ , ์ฝ๋
Target Interface
→ Adapter๊ฐ implementsํ๋ ์ธํฐํ์ด์ค
- ํด๋ผ์ด์ธํธ๋ Target Interface๋ฅผ ํตํด Adaptee๋ฅผ ์ฌ์ฉํ ์ ์๊ฒ ๋๋ค.
Adapter
→ ํด๋ผ์ด์ธํธ์ Adaptee๋ฅผ ์ฐ๊ฒฐ์์ผ์ฃผ๋ Adapter
- ํด๋ผ์ด์ธํธ์ ์์ฒญ์ Adaptee๊ฐ ์ดํดํ ์ ์๋ ๋ฐฉ๋ฒ์ผ๋ก ์ ๋ฌ
- Adaptee ํด๋์ค๋ฅผ Target Interface์ ๋ง์ถ์ด ์ ๊ณต
Class Adapter Pattern
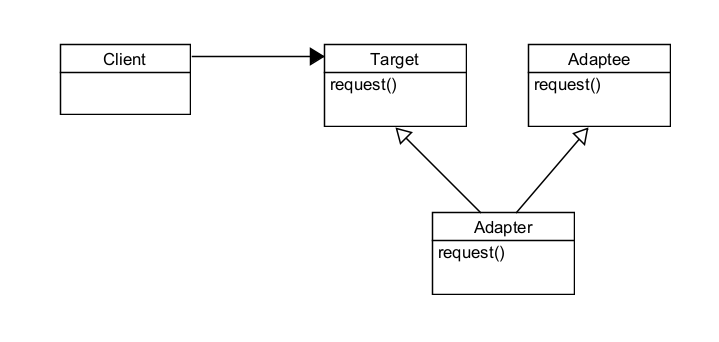
ํด๋์ค ์ด๋ํฐ๋ ๋ค์ค ์์์ ์ง์ํ์ฌ Adapter๊ฐ Target ํด๋์ค์ Adaptee ํด๋์ค๋ฅผ ๋ ๋ค ์์ํ๋ค.
๐ก Practice Adapter Pattern
Object Adapter Pattern
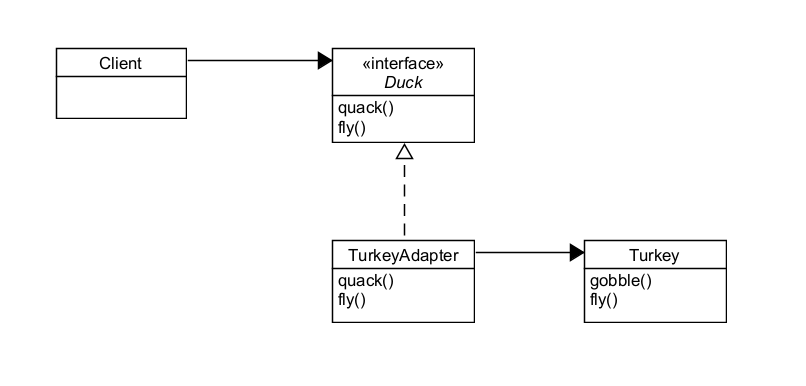
- Duck
package Adapter;
public interface Duck {
public void quack();
public void fly();
}
- Turkey
package Adapter;
public interface Turkey {
public void gobble();
public void fly();
}
- TurkeyAdapter
Turkey๊ฐ์ฒด๋ฅผ ์ฌ์ฉํ์ฌ Turkey์ gooble๋ฉ์๋๋ฅผ Duck์ quack๋ฉ์๋๋ก ๋ง์ถ์ด์ค๋ค.
package Adapter;
public class TurkeyAdapter implements Duck{
Turkey turkey;
public TurkeyAdapter(Turkey turkey){
this.turkey = turkey;
}
@Override
public void quack() {
turkey.gobble();
}
@Override
public void fly() {
for(int i = 0; i < 5; i++){
turkey.fly();
}
}
}
- Main
TurkeyAdapter๋ฅผ ํตํด Duck interface๊ฐ์ฒด๋ก Turkey๋ฅผ ์์ฑํด์ค๋ค.
public class Main {
public static void main(String[] args) {
Duck turkey = new TurkeyAdapter(new Turkey());
System.out.println("I am a duck");
duck.quack();
duck.fly();
System.out.println("I am a turkey");
turkey.quack();
turkey.fly();
}
}
Class Adapter Pattern
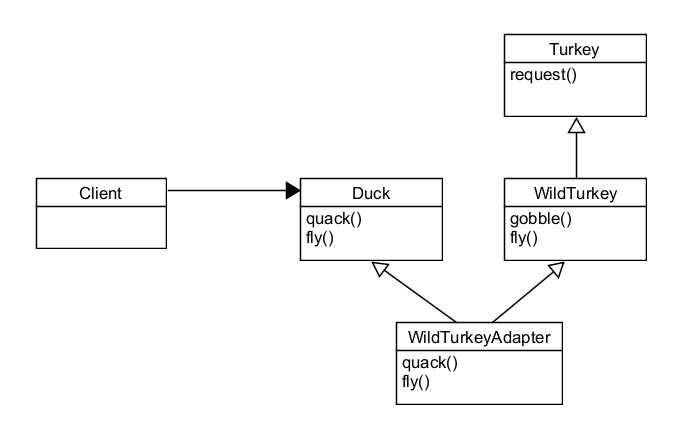
- WildTurkeyAdapter
Duck ์ธํฐํ์ด์ค์ WildTurkey ํด๋์ค๋ฅผ ๋ชจ๋ ์์๋ฐ๋ WildTurkeyAdapter
package Adapter;
public class WildTurkeyAdapter extends WildTurkey implements Duck{
@Override
public void quack() {
gobble();
}
@Override
public void fly() {
for (int i = 0; i < 5; i++){
super.fly();
}
}
}
- Main
package Adapter;
public class Main {
public static void main(String[] args) {
Duck turkey = new WildTurkeyAdapter();
System.out.println("I am a duck");
duck.quack();
duck.fly();
System.out.println("I am a turkey");
turkey.quack();
turkey.fly();
}
}
Object Adapter๋ ์์์ด ์๋ ๊ตฌ์ฑ์ ์ฌ์ฉํ๊ธฐ ๋๋ฌธ์ Adaptee์ ๋ํ ๋ชจ๋ ์๋ธํด๋์ค์ ์ด๋ํฐ๋ฅผ ์ ์ฉํ ์ ์๋ ๋ฑ ๋น๊ต์ ์ ์ฐํ๊ฒ ์ฌ์ฉ์ด ๊ฐ๋ฅํ ๋ฐ๋ฉด, Class Adapter๋ Adapter๊ฐ Adaptee์ ์๋ธํด๋์ค์ด๊ธฐ ๋๋ฌธ์ Adaptee๋ฅผ ์ค๋ฒ๋ผ์ด๋ ํ ์ ์๊ณ , Main์ฝ๋์์ ๋ณผ ์ ์๋ฏ Adaptee ๊ฐ์ฒด๋ฅผ ๋ง๋ค์ง ์์๋ ๋๋ค๋ ์ฅ์ ์ด ์๋ค๐ค๐ค
'Software > Design Pattern' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[Design Pattern] Command Pattern (4) | 2021.11.28 |
---|---|
[Design Pattern] Observer Pattern (2) | 2021.10.08 |